Traditionally, Microsoft SharePoint list data is filtered using CAML query. But when querying the same SharePoint list data from Microsoft flow, CAML query will not work because Microsoft flow was built on top of Logic apps. So, to query the SharePoint list data in Microsoft Flow, OData filter expressions need to be used instead of CAML query. Get SharePoint Items By CAML Query is the action from Plumsail SharePoint connector. You can use it to get various information about items or documents by using CAML query. Apr 11, 2015 CAML QUERY to retrieving the items from a list using CSOM Next Recommended Reading Update A List Item In SharePoint Using CSOM (Announcement List).
- Filtering List Item using CAML query in sharepoint. By rautchetan27 on May 25, 2020. 1504 Views 0 Likes. Related Videos View all.
- In this article, we will discuss how to query a SharePoint List using CAML in SharePoint 2010. SPQuery object has Query property that accepts a CAML fragment, which defines the query to be performed. A ViewFields property defines the fields to return.
Creating the Lists
Querying Using JOINS and SPQuery
Querying Using LINQ
Using Caml Query Sharepoint List Template
Sharepoint Caml Query Tutorial
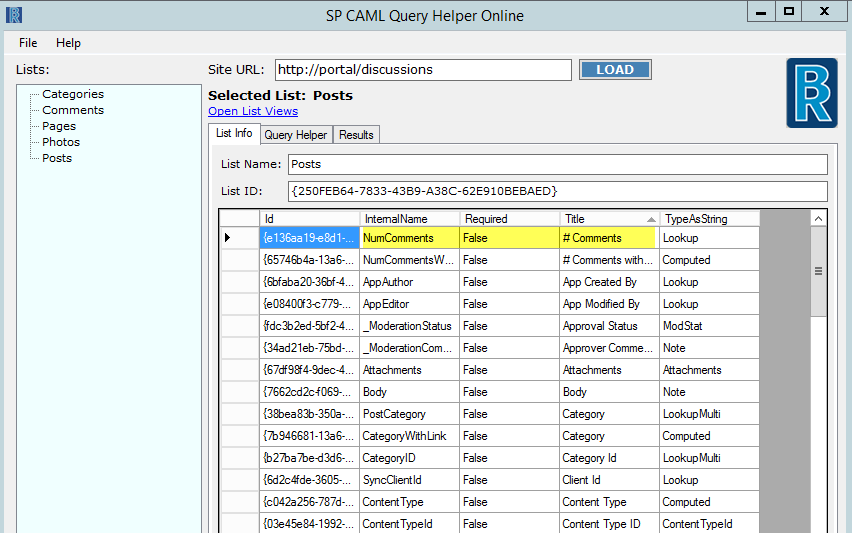
Sharepoint Caml Query Examples
I’ve been doing a lot of work with SPServices and SharePoint lists lately, and I find myself using the same CAML queries over and over. Unfortunately I don’t always remember how to format some of the more common queries, so I decided to make a quick reference.
There are already some good tools and resources out there for building CAML queries. U2U’s CAML Query Builder is usually what I fall back to when I need a complex query with multiple conditions. Matt Bramer’sroboCAML is shaping up very nicely as well. However, in many cases these are overkill and I just need a basic “get all items where field X is equal to value Y” query. Heck, sometimes I just want to hard-code the query myself because it’s good practice. A quick reference that shows what value type to use and in what format for the various field types is all I really need so I don’t have to hunt through several bookmarked articles to find the answer.

I intend to update this on occasion, so if you have any suggestions please leave them in the comments.
Column/Field Type | Value Type | Example | Notes |
---|---|---|---|
Single Line of Text | Text | This is one of the simplest queries. The example selects items with a title equal to “Hello World!” | |
Multiple Lines of Text | Text | If this is a Rich Text field, you can use <![CDATA[]]> around the value to prevent parsing errors when passing HTML into the query. Alternatively, you can encode the HTML by replacing < with < , > with > , and ' with " . This query uses <Contains> to return any items that contain a hyperlink in the body field by looking for the closing </a> tag. | |
Person or Group (By Name) | Text | This will look for items created by any user with “Josh McCarty” in the Name field of the User Information list. If more than one person has the same display name in the user list, it will select items created by all users with that name. | |
Person or Group (By ID) | Integer | By adding LookupId='TRUE' to the <FieldRef /> and using <UserID /> as the value, the query will filter based on the current user. You can also pass the ID of a specific user in place of <UserID /> (e.g. <Value Type='Integer'>283</Value> ) if you don’t want to filter by the current user. IDs are always unique, so this method ensures that only one user is a valid value. | |
Lookup (By Text) | Lookup | This will look for items with “Arizona” in the State field. If more than one state has the same display name (not likely in this example, but for other lookups it could happen), it will return items from all states with that display name. | |
Lookup (By ID) | Lookup | By adding LookupId='TRUE' to the <FieldRef /> , the query will filter based on the ID of the lookup rather than the text value. IDs are always unique, so this method ensures that only one state is a valid value. | |
Date (Day Only) | Date | This type of query seems to work whether the value type is set to DateTime or just Date as long as the value is formatted properly (yyyy-mm-dd). It also works if <Today /> is used as the value (you can offset the current date; e.g. use <Today OffsetDays='-7' /> for 7 days ago). See one of my previous posts regarding current date offsets for some more information about using <Today /> . | |
ContentTypeId | ContentTypeId | This is useful for querying items with a specific content type or, if using <BeginsWith> , items with a specific content type or child content types. You can get the content type ID in the web UI by viewing that content type from the site collection or list content types (it should be in the URL). |
Caml Query Sharepoint List Id
I’ll be adding more column types to this post as I have time to write them, and I’ll probably create a PDF once they are all finished. For now I wanted to get this published sooner rather than later. If you have any suggestions or sample queries, leave them in the comments!
